React.JS + MSAL with ASP.NET Core to use Azure AD with User & Role - Part 1
React.JS + MSAL with ASP.NET Core to use Azure AD with User & Role - Part 3
1. Open the Visual Studio and choose the web api application. I'm using net6.0
framework but you can use the older version of .net core.
Microsoft.Identity.Web
2.1 Go to the nuget package manager and search for Microsoft.Identity.Web
and install.
Alternatively, you can use the .NET CLI version which is the: dotnet add package Microsoft.Identity.Web
appsettings.json
for the AzureAD
key. Here's the sample reference.
{
"AzureAd": {
"scopes": "api.scope", // CREATED from the App Registration for WebAPI/Expose an API
"ClientId": "PUT_YOUR_CLIENT_ID_HERE", // Client ID of the web api from App Registration
"Instance": "https://login.microsoftonline.com",
"TenantId": "PUT_YOUR_TENANT_ID_HERE" // Tenant ID of the web api from App Registration
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*"
}
As you can see, we also included our scope
because we will need it in our controller.
Startup.cs
4.1 Add Authentication
to the container
using Microsoft.AspNetCore.Authentication.JwtBearer;
using Microsoft.Identity.Web;
...
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(builder.Configuration);
4.2 Register CORS
policy for our client app inside the container.
builder.Services.AddCors(options => options.AddPolicy("CorsPolicy", policy =>
{
policy.AllowAnyHeader().AllowAnyMethod().AllowCredentials().WithOrigins("http://localhost:3000");
}));
CORS
and to use Authentication and Authorization. 5.1 Add this below the app.UseHttpsRedirection();
app.UseCors("CorsPolicy");
app.UseAuthentication();
app.UseAuthorization();
6.1 Add this above your controller name.
[RequiredScope(RequiredScopesConfigurationKey = "AzureAd:scopes")]
6.2 Authorize the endpoints.
[Authorize(Roles = "Admin")]
[HttpGet("employees")] // Add this
public IActionResult GetEmployees()
{
return Ok(Employees);
}
[Authorize] // Add this
[HttpGet("total-employees")]
public IActionResult TotalEmployees()
{
return Ok(Employees.Length);
}
In the example, we have an endpoint that allows the access only if the Azure AD user has an Admin role. We also have the [Authorize]
without the role assigned, this means that regardless of the role, as long as the Azure AD user is part of the user inside the app registration for the web api, then the user will have an access.
You can see the reference below.
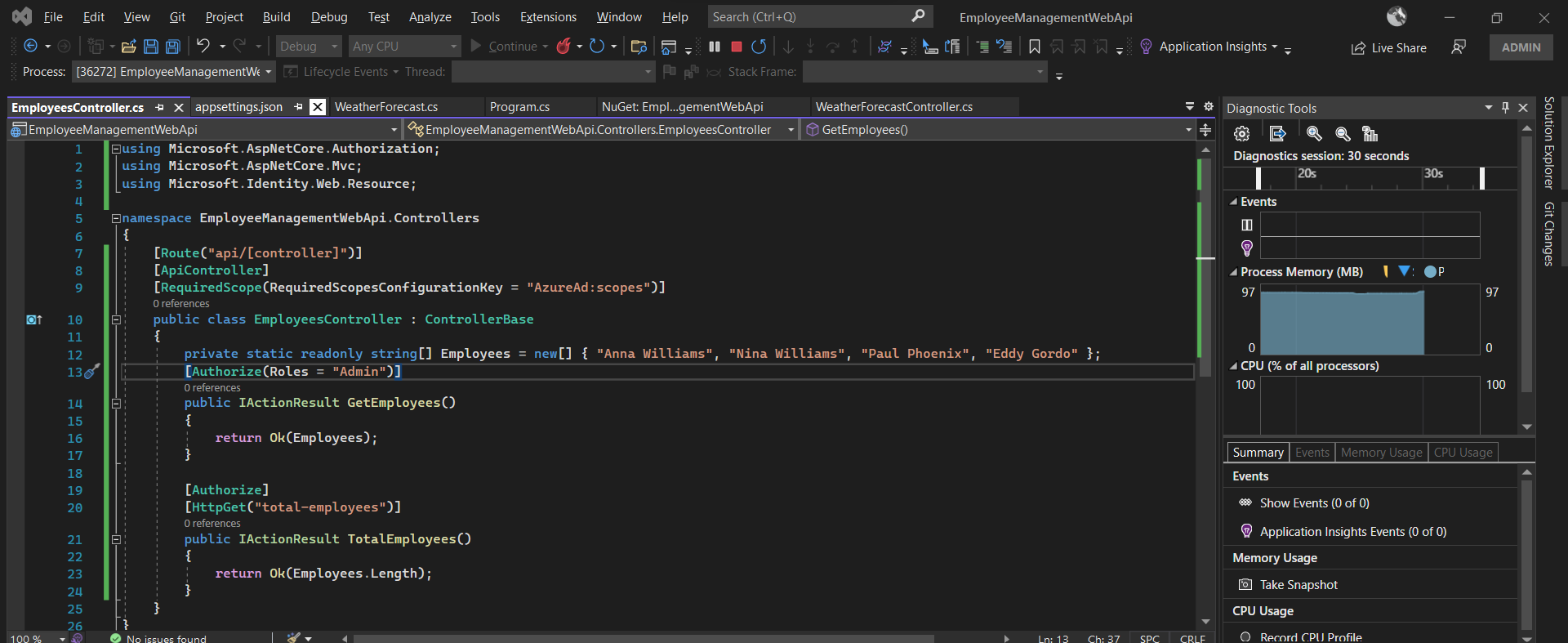
Let's test using postman if the endpoints are already authorize.
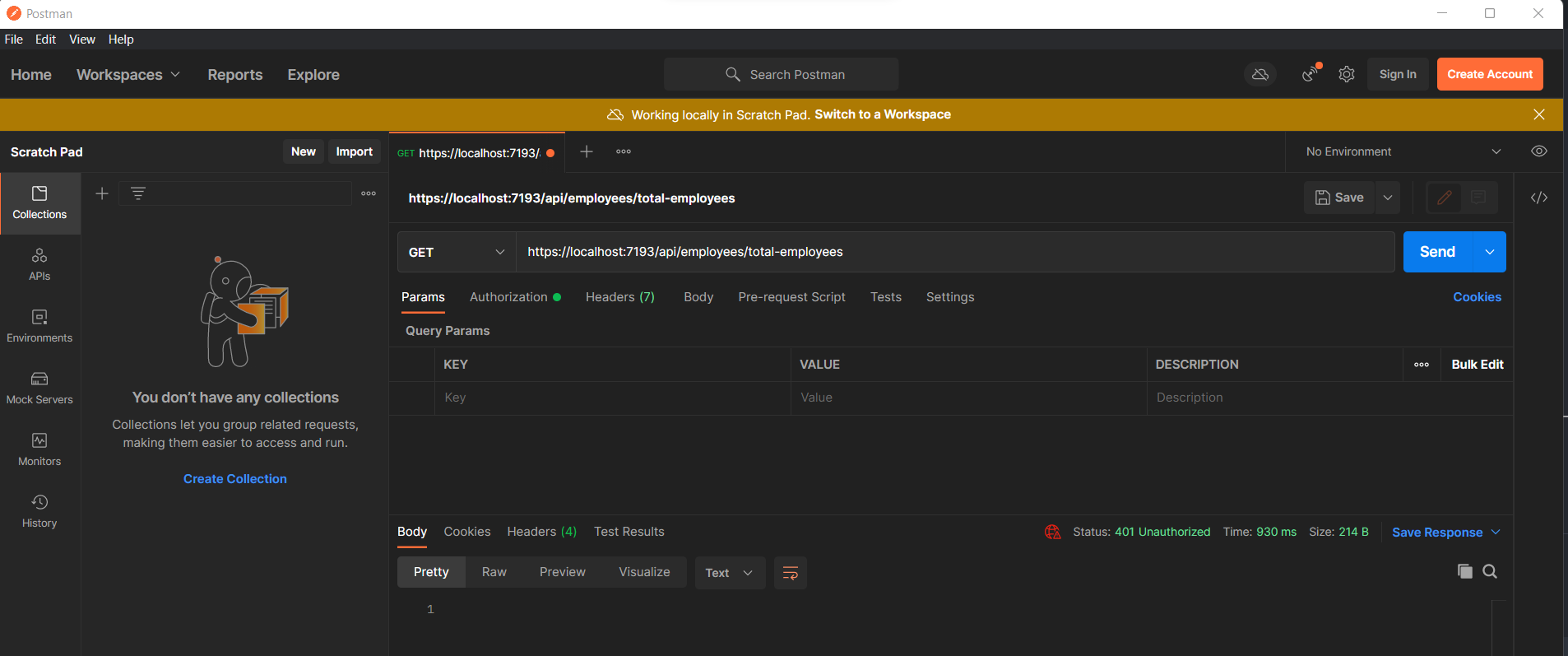
As expected, we receive the 401 Unauthorized result.
On this blog, we worked on setting up our ASP.NET Core Web API solution, install necessary package and configure the app to properly authenticate and authorize the application. On the last part, we'll setup our frontend to communicate or connect with our backend solution.