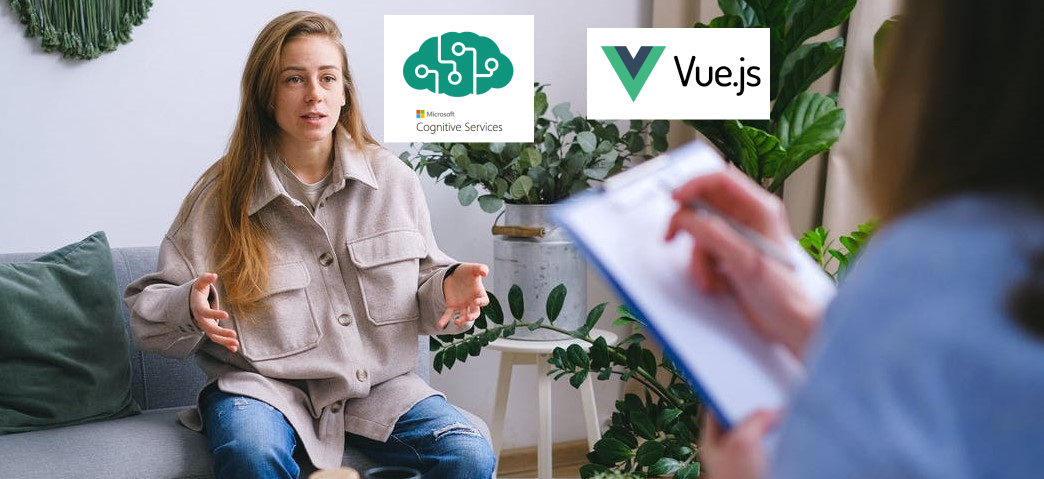
In my previous blog post with a title: Integrate Azure Cognitive Services Speech to Text to your React.JS App, I already wrote an introduction regarding the "Speech-to-text" and how it helps people nowadays. But in case you want to read the advantages of using speech-to-text, I've added them below for your reference.
Here are the advantages of the Speech-to-Text:
- * Ease of Communication - No more or lessen the illegible of typing or writing.
- * Increase Efficiency & Less Paperwork
- * Can Produce Faster Documents
- * It Solves Inefficiencies and Reduces Wasted Time
- * Flexibility to Share Across Different Devices and more
Truly, the technologies are evolving and innovations are keep on moving up. One of the modern improvements is the Cognitive Services. These services are available via subscriptions from the top companies like Microsoft, Google and Amazon.
For this blog, we'll be using Azure Cognitive Services to integrate the Speech to Text to our Vue.JS.
You can visit the official documentation from Microsoft Docs Speech-to-Text Cognitive Services to learn more.1. Machine with your text editor/IDE
2. Microsoft Azure Account (Try it for free)
3. Vue.JS Application
1.1 Create resource in Azure Portal. (Make sure you already have subscription whether free or paid in Azure)
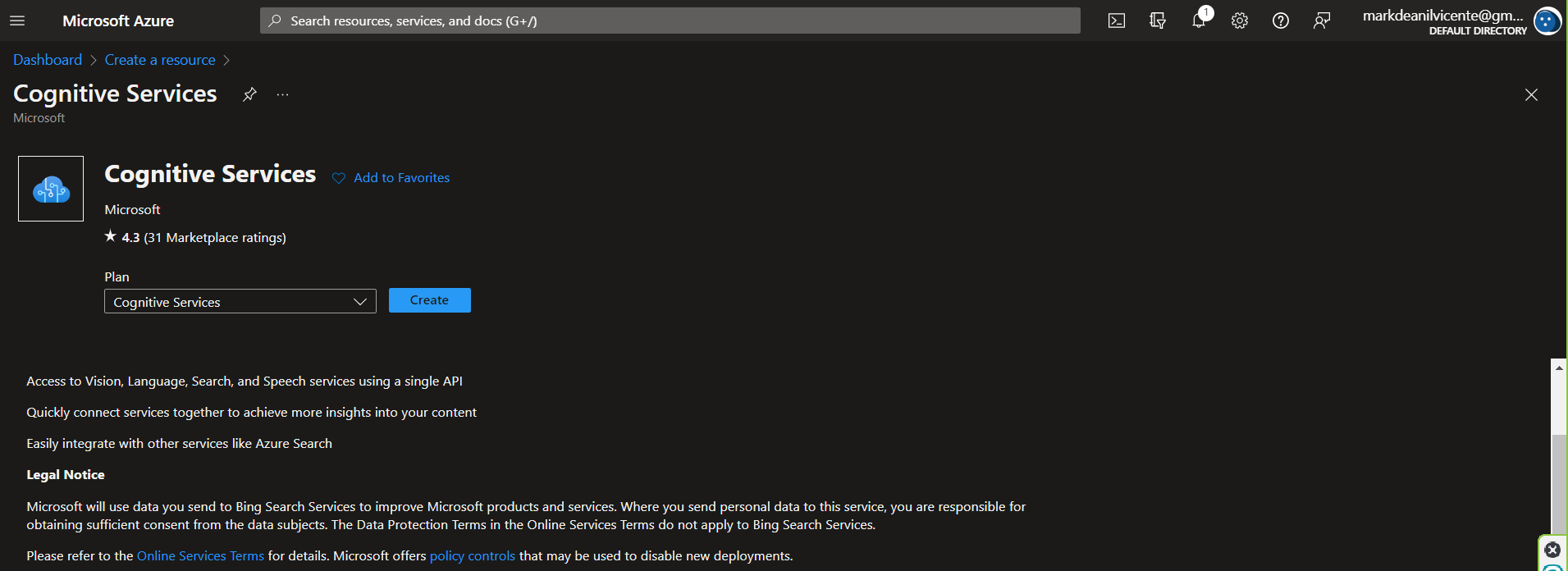
Below is a sample. Click the "Create" then once the creation is done, click the button "Go to resource"
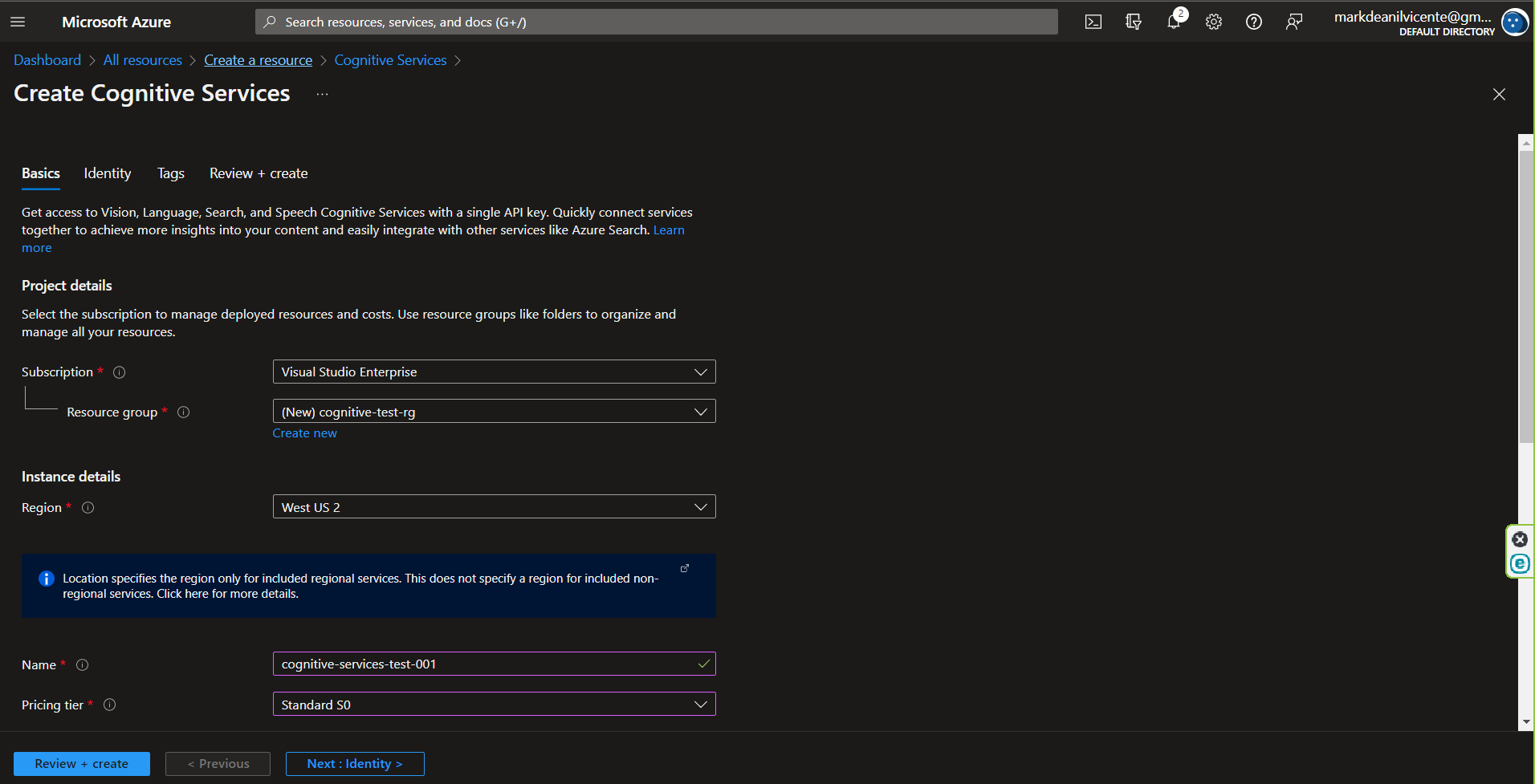
1.2 click the "Click here to manage keys" to navigate to the key section.
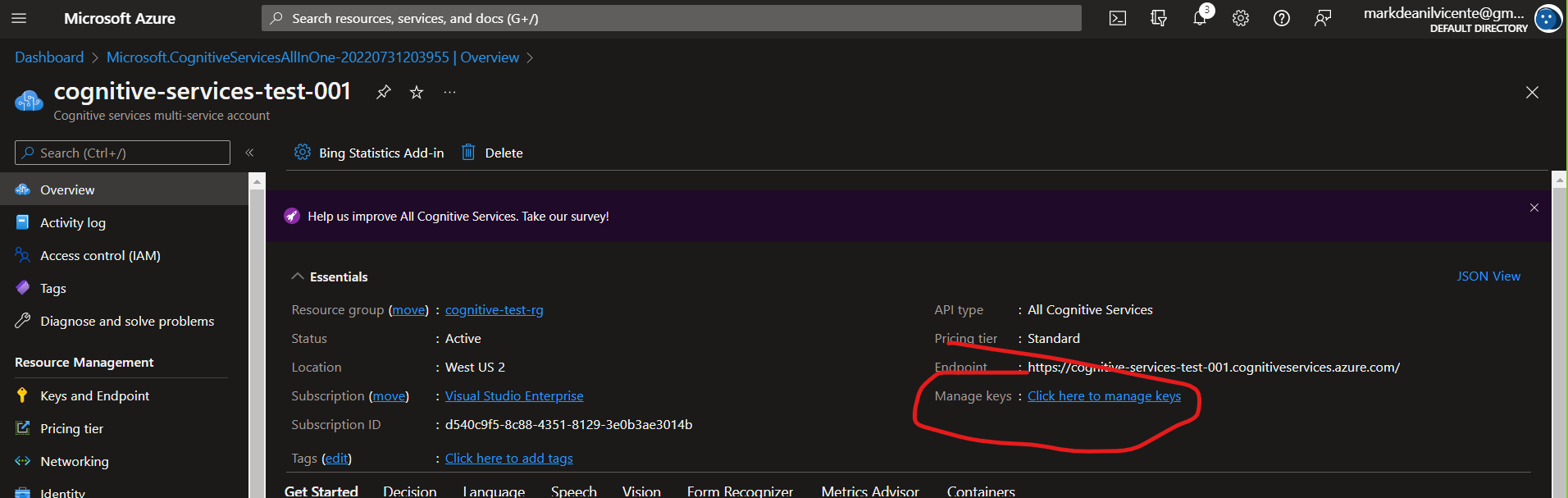
1.3 Save the keys because we are going to need them on our vue.js configuration.
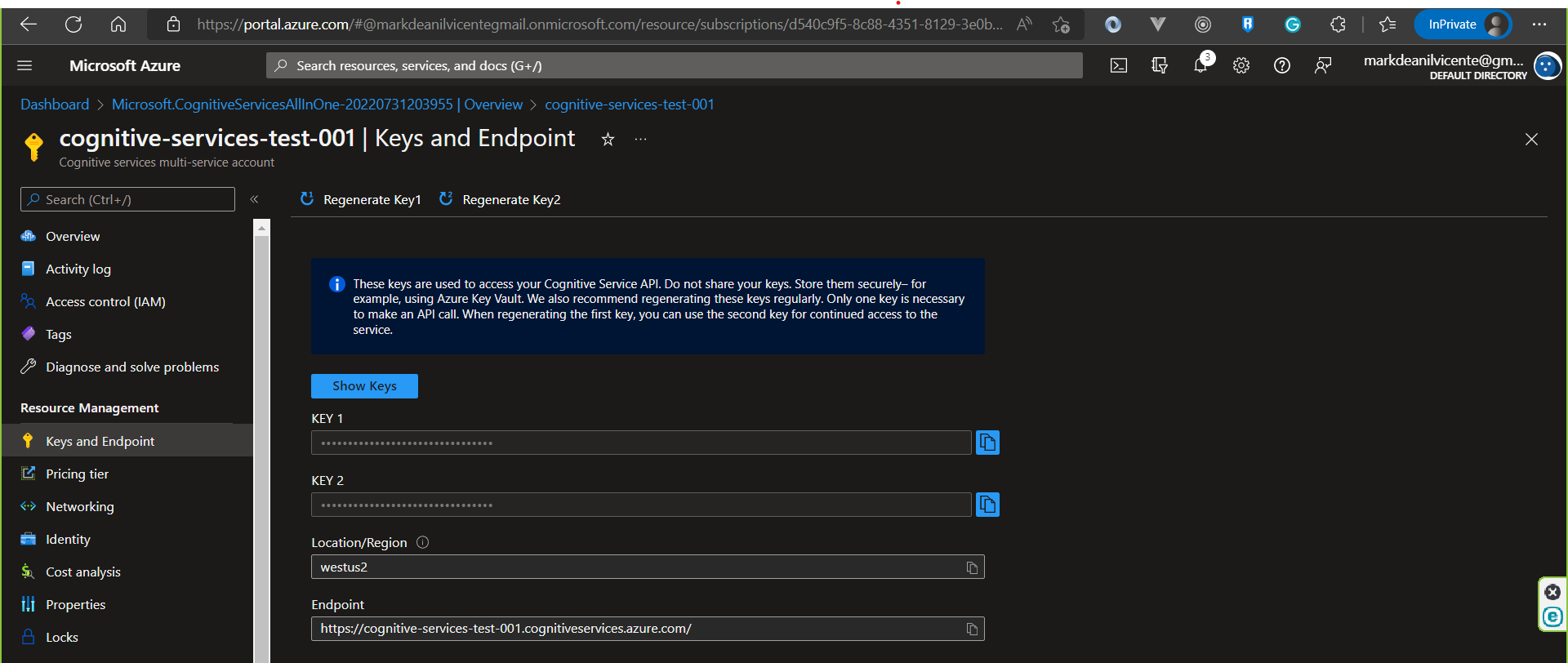
2.1 npm i microsoft-cognitiveservices-speech-sdk
On my sample, I'm using the Vue.JS project template. For this demo, I'm overwriting the App.tsx
file.
3.1 Import the package below.
const sdk = require("microsoft-cognitiveservices-speech-sdk");
3.2 Inside your component function, configure the speech sdk
const key = "YOUR_KEY_FROM_YOUR_COGNITIVE_SERVICE";
const region = "westus2";
const speechConfig = sdk.SpeechConfig.fromSubscription(key, region);
// Create the speech recognizer.
let speechRecognizer = new sdk.SpeechRecognizer(speechConfig);
3.3 Create a function that invoke the recognizeOnceAsync
from the SDK's Synthesizer
const speechToText = () => {
speechRecognizer.recognizeOnceAsync((result) => {
switch (result.reason) {
case sdk.ResultReason.RecognizedSpeech:
text.value(result.text);
break;
case sdk.ResultReason.NoMatch:
console.log("NOMATCH: Speech could not be recognized.");
break;
case sdk.ResultReason.Canceled:
const cancellation = sdk.CancellationDetails.fromResult(result);
console.log(`CANCELED: Reason=${cancellation.reason}`);
if (cancellation.reason === sdk.CancellationReason.Error) {
console.log(`CANCELED: ErrorCode=${cancellation.ErrorCode}`);
console.log(`CANCELED: ErrorDetails=${cancellation.errorDetails}`);
console.log(
"CANCELED: Did you set the speech resource key and region values?"
);
}
break;
}
speechRecognizer.close();
});
};
3.4 Call the function inside the useEffect
or create an UI that has a button and input that trigger the function.
Below is a sample code that you paste and play around on your machine.
<template>
<div>
<input v-model="text" />
<button @click="speechToText()">Run Speech to Text</button>
</div>
</template>
<script setup>
// call the package
import { ref } from "vue";
import * as sdk from "microsoft-cognitiveservices-speech-sdk";
const key = "YOUR_KEY_FROM_YOUR_COGNITIVE_SERVICE";
const region = "westus2";
const speechConfig = sdk.SpeechConfig.fromSubscription(key, region);
// Create the speech recognizer.
let speechRecognizer = new sdk.SpeechRecognizer(speechConfig);
const text = ref("");
const speechToText = () => {
speechRecognizer.recognizeOnceAsync((result) => {
switch (result.reason) {
case sdk.ResultReason.RecognizedSpeech:
text.value(result.text);
break;
case sdk.ResultReason.NoMatch:
console.log("NOMATCH: Speech could not be recognized.");
break;
case sdk.ResultReason.Canceled:
const cancellation = sdk.CancellationDetails.fromResult(result);
console.log(`CANCELED: Reason=${cancellation.reason}`);
if (cancellation.reason === sdk.CancellationReason.Error) {
console.log(`CANCELED: ErrorCode=${cancellation.ErrorCode}`);
console.log(`CANCELED: ErrorDetails=${cancellation.errorDetails}`);
console.log(
"CANCELED: Did you set the speech resource key and region values?"
);
}
break;
}
speechRecognizer.close();
});
};
</script>
You can read the official documentation from Microsoft where you can learn more about real-time speech-to-text, batch speech-to-text and the custom speech. Speech-to-text documentation