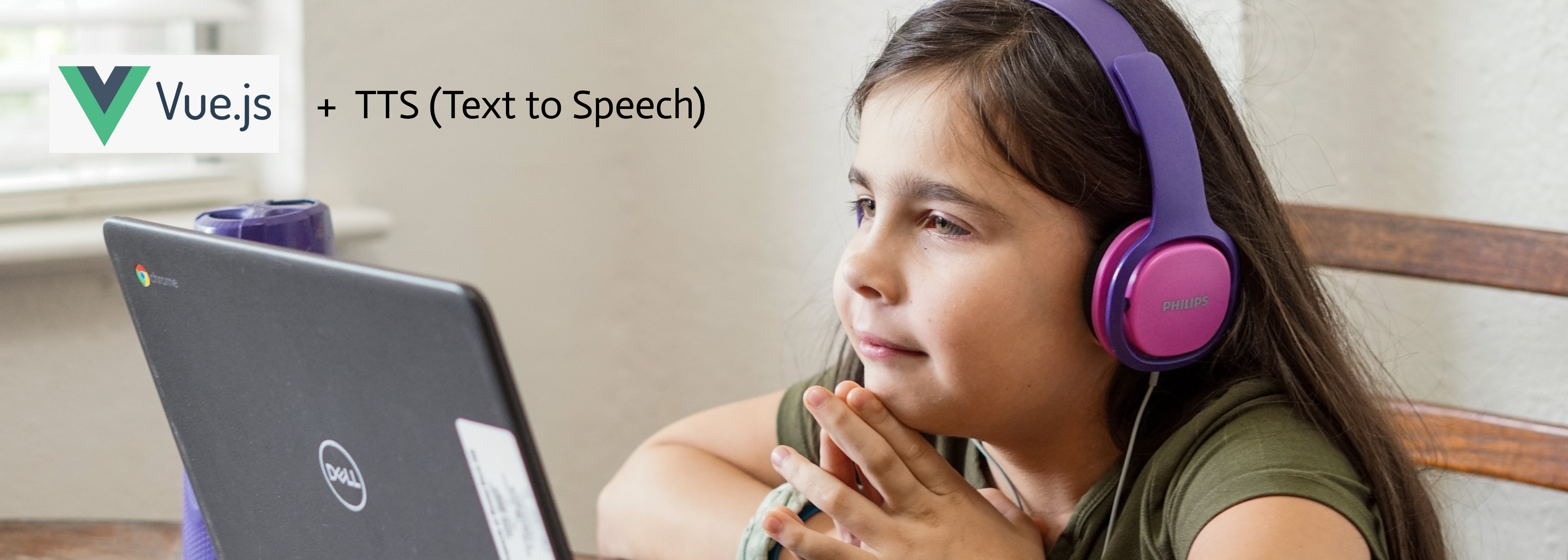
Related post: Integrate Azure Cognitive Services Text to Speech to your Vue.JS App
Text-to-speech or TTS is a type of assistive technology that reads certain digital text aloud. This technology brings a lot of benefits in different industries, whether in teaching, announcement, broadcasting, entertainment and even to you when you're bored reading certain text. Why not just listen to them instead?
This technology not only gives us efficiency in the majority, but it also beneficial to those people with literacy difficulties, learning disabilities, reduced vision and those learning a language.
These are the top cloud providers that offer Text-to-speech API: Microsoft Azure Text to speech, Google Cloud Text-to-Speech & Amazon Text-to-Speech. Other cloud services also offer this service, but it depends on you what will you choose.
But in this blog, we'll be using Microsoft Azure Cognitive Services Text to speech. Without further ado, let's start coding!
1. Machine with your text editor/IDE
2. Microsoft Azure Account (Try it for free)
3. Vue.JS Application
1.1 Create resource in Azure Portal. (Make sure you already have subscription whether free or paid in Azure)
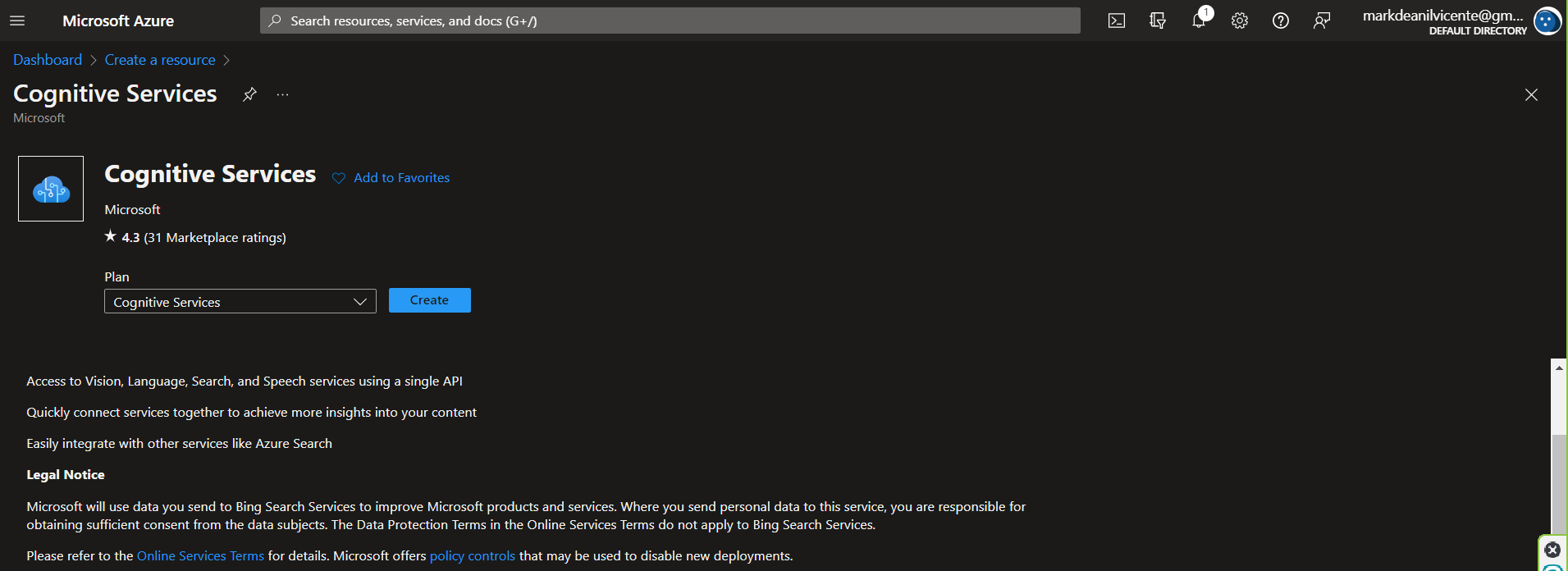
Below is a sample. Click the "Create" then once the creation is done, click the button "Go to resource"
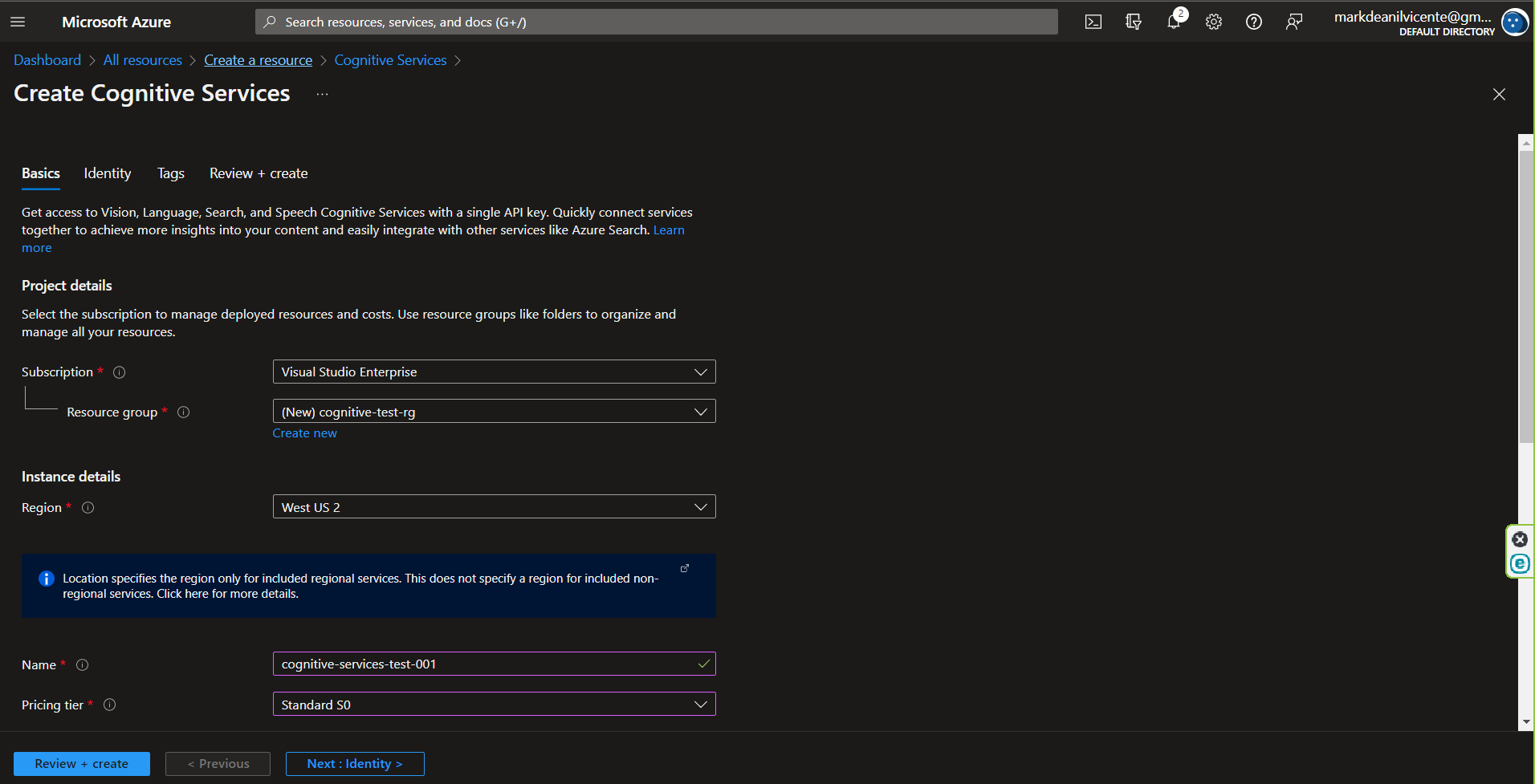
1.2 click the "Click here to manage keys" to navigate to the key section.
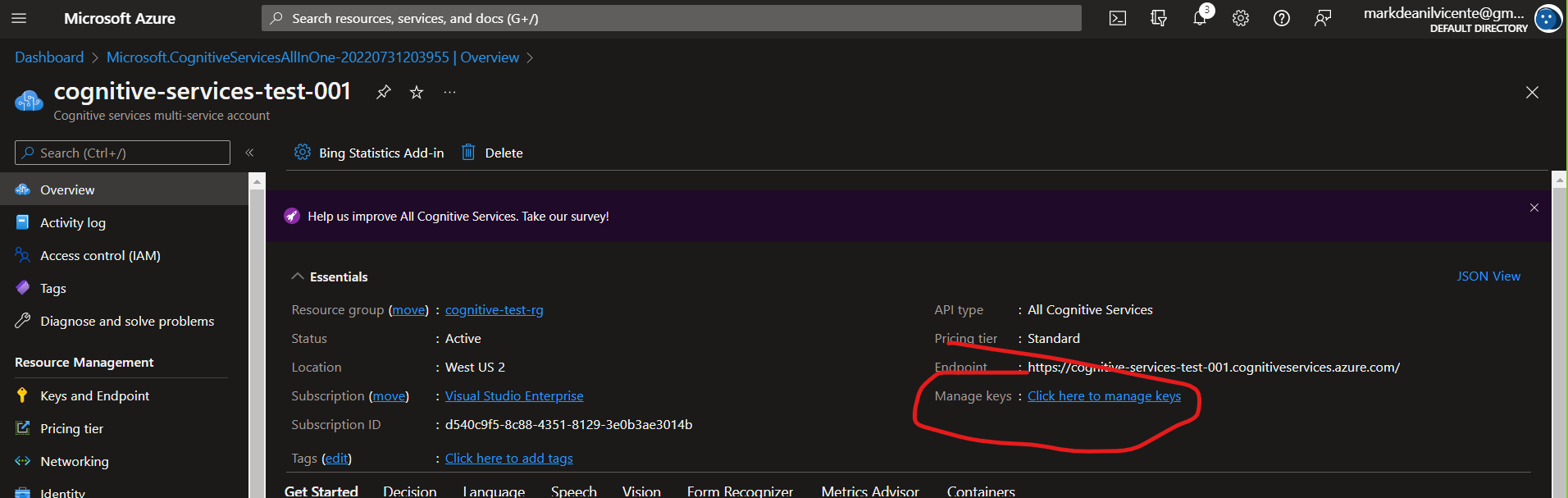
1.3 Save the keys because we are going to need them on our Vue.js configuration.
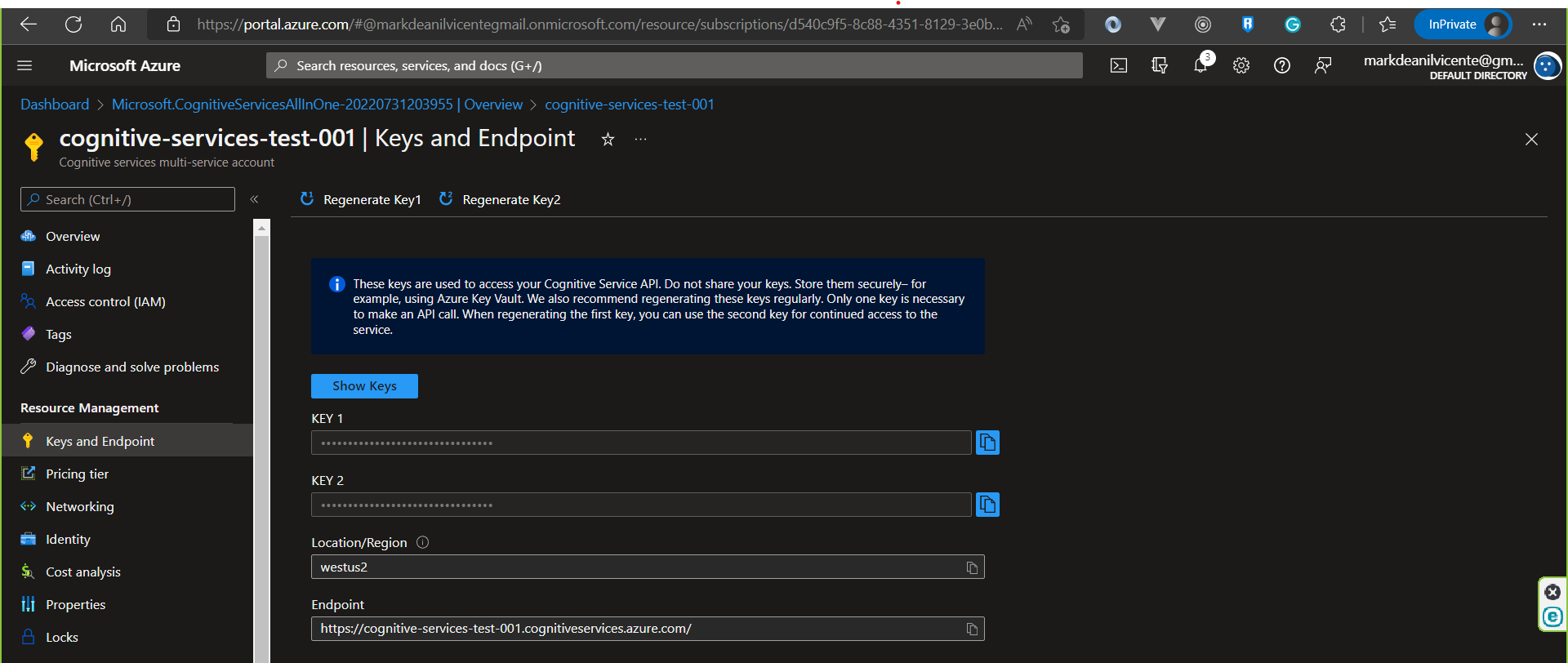
2.1 npm i microsoft-cognitiveservices-speech-sdk
On my sample, I'm using the Vue.JS with Composition API project template. For this demo, I'm overwriting the App.vue
file.
3.1 Import the package below.
import * as sdk from "microsoft-cognitiveservices-speech-sdk"
3.2 Inside the script, configure the speech sdk
// Text to Speech Config
const key = "YOUR_KEY_FROM_YOUR_COGNITIVE_SERVICE";
const region = "westus2";
const speechConfig = sdk.SpeechConfig.fromSubscription(key, region);
// The language of the voice that speaks.
speechConfig.speechSynthesisVoiceName = "en-US-JennyNeural";
3.3 Create a function that invoke the speakTextAsync
from the SDK's Synthesizer
const textToSpeech = () => {
let synthesizer = new sdk.SpeechSynthesizer(speechConfig);
synthesizer.speakTextAsync(
"Enter your text here.",
function (result) {
if (result.reason === sdk.ResultReason.SynthesizingAudioCompleted) {
console.log("synthesis finished.");
} else {
console.error(
`Speech synthesis canceled: ${result.errorDetails}. Did you set the speech resource key and region values?`
);
}
synthesizer.close();
synthesizer = null;
isLoading.value = false;
},
function (err) {
isLoading.value = false;
console.trace(`Error: ${err}`);
synthesizer.close();
synthesizer = null;
}
);
};
3.4 Call the function from your UI and trigger the textToSpeech()
function.
Below is a sample code that you paste and play around on your machine.
<template>
<div>
<input v-model="text" />
<p v-if="isLoading">Loading...</p>
<button v-else @click="textToSpeech()">Run Text to Speech</button>
</div>
</template>
<script setup>
// call the package
import { ref } from "vue";
import * as sdk from "microsoft-cognitiveservices-speech-sdk";
// Text to Speech Config
const key = "YOUR_KEY_FROM_YOUR_COGNITIVE_SERVICE";
const region = "westus2";
const speechConfig = sdk.SpeechConfig.fromSubscription(key, region);
// The language of the voice that speaks.
speechConfig.speechSynthesisVoiceName = "en-US-JennyNeural";
const text = ref("");
const isLoading = ref(false);
const textToSpeech = () => {
if (text.value) {
// Create the speech synthesizer.
let synthesizer = new sdk.SpeechSynthesizer(speechConfig);
isLoading.value = true;
synthesizer.speakTextAsync(
text.value,
function (result) {
if (result.reason === sdk.ResultReason.SynthesizingAudioCompleted) {
console.log("synthesis finished.");
} else {
console.error(
`Speech synthesis canceled: ${result.errorDetails}. Did you set the speech resource key and region values?`
);
}
synthesizer.close();
synthesizer = null;
isLoading.value = false;
},
function (err) {
isLoading.value = false;
console.trace(`Error: ${err}`);
synthesizer.close();
synthesizer = null;
}
);
}
};
</script>
You can change the speechSynthesisVoiceName
or speech voice from this complete list.
You can read more about this API from this documentation.